How to add dropdowns to textboxes in HTML5
I recently had to add a dropdown box to a textbox at work, instead of jumping on the first jQuery plugin I could find, I instead started checking if this feature was finally added to "native html", and it was, and it is called a datalist.
Simple Example
A datalist in HTML5 is a simple way giving a (textbox) input field a dropdown of choices to select from, you bind a
datalist and an input field via using the list="magic-ponies"
attribute on the input field like so:
<input list="hosting-plan" type="text" />
<datalist id="hosting-plan">
<option value="small" />
<option value="medium" />
<option value="large" />
</datalist>
which will give you this:
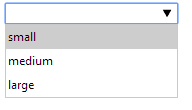
Simple example with additional text
As you can see the way you specify values inside the datalist is very similar to how you would populate a <select>
box, using the <option value="blah">
, except that you can choose to only have a single self-closing option tag instead
of the usual option-pair like <option value="blah">foo</option>
.
The neat thing about the datalist tag is that if you do choose to give it a text inside the option tag like with a select box, like this:
<input list="hosting-plan" type="text" />
<datalist id="hosting-plan">
<option value="small">$15 USD</option>
<option value="medium">$20 USD</option>
<option value="large">$25 USD</option>
</datalist>
Your textbox will look like this, notice that the price is right-aligned, veryfancy.
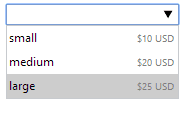
Dynamically add list items So all you need to dynamically add items into thedatalist is to append an
<option>
element within the
<datalist>
with some simple JavaScript.
<input list="hosting-plan" type="text" />
<datalist id="hosting-plan">
<option value="small">$10 USD</option>
<option value="medium">$20 USD</option>
<option value="large">$25 USD</option>
</datalist>
<script>
// Create a new option element. var optionNode =
document.createElement("option"); // Set the value optionNode.value = "huge";
// create a text node and append it to the option element
optionNode.appendChild(document.createTextNode("$50 USD")); // Add the
optionNode to the datalist
document.getElementById("hosting-plan").appendChild(optionNode);
</script>
</datalist>
</option>
Populating list dynamically from external resource Here is an example
for dynamically adding data into a datalist from an online resource, in this case the public reddit JSON "file" containing a list of subreddits (http://www.reddit.com/subreddits.json), I am going to display the name of the subreddit and the amount of subscribers of that subreddit in the datalist.
<html>
<head>
<title>Subreddit Picker</title>
</head>
<body>
<h1>Select your favorite Subreddit</h1>
<input type="text" list="hosting-plan" />
<datalist id="hosting-plan"></datalist>
<script>
// Wait for the dom to be loaded
document.addEventListener('DOMContentLoaded', function (event) {
// Setup an XMLHttpRequest / AJAX request
var request = new XMLHttpRequest();
request.open('GET', 'http://www.reddit.com/subreddits.json');
// Setup an "event listener".
request.onload = function () {
if (request.status >= 200 && request.status < 400) {
var response = JSON.parse(request.responseText.toString());
response.data.children.forEach(function (el) {
addListEntry(el.data.display_name, "Subs: " + el.data.subscribers);
});
}
};
// Send our request
request.send();
});
// Break the list adding code into a function for easier re-use
function addListEntry(value, text) {
// Create a new option element.
var optionNode = document.createElement("option");
// Set the value
optionNode.value = value;
// create a text node and append it to the option element
optionNode.appendChild(document.createTextNode(text));
// Add the optionNode to the datalist
document.getElementById("hosting-plan").appendChild(optionNode);
}
</script>
</body>
</html>
</datalist>
</option>
You can check out the example site here.
Browser Support
As web developers we often have to maintain support for older browsers and systems, I fortunately only have to officially support Chrome and the Default Android and iOS browsers at my workplace, but for the completeness, here are a breakdown of the browser support for the datalist element.
- Internet Explorer
- Partially supported by IE from version 10
- Not supported before version 9
- FireFox
- Supported from version 4
- Chrome
- Supported from version 20
More detailed information can be found on Can I Use.
I hope you learned something new from this post, now go forth and build awesome HTML5 thingies.
Note: To better support older browsers and Safari, you can include this polyfill.
Update 25.11.2015
Webucator was so kind as to use this blog post as an inspiration for one of their videos, you can watch the video below.
https://www.youtube.com/watch?v=iNRQm15qtg8
Webucator is a company that provides various training courses related to web development and various other tech fields, feel free to check out their HTML Training as well as their other courses.